-
Notifications
You must be signed in to change notification settings - Fork 0
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
- Loading branch information
1 parent
6836a7a
commit 7413759
Showing
8 changed files
with
196 additions
and
70 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -1,15 +1,85 @@ | ||
# nats | ||
# NATS | ||
NATS is an open-source, lightweight, and high-performance messaging system designed for cloud-native applications, IoT messaging, and microservices architectures. It supports multiple messaging patterns, including publish/subscribe, request/reply, and queuing. Key features include: | ||
- <b>Simplicity</b>: Easy to set up and use with minimal configuration. | ||
- <b>Performance</b>: Low latency and high throughput. | ||
- <b>Scalability</b>: Capable of handling millions of messages per second. | ||
- <b>Fault Tolerance</b>: Supports clustering for high availability. | ||
### Libraries for Google Pub/Sub | ||
- GO: [NATS](https://github.com/core-go/nats). Example is at [go-nats-sample](https://github.com/project-samples/go-nats-sample) | ||
- nodejs: [NATS](https://github.com/core-ts/nats). Example is at [nats-sample](https://github.com/typescript-tutorial/nats-sample) | ||
|
||
#### A common flow to consume a message from a message queue | ||
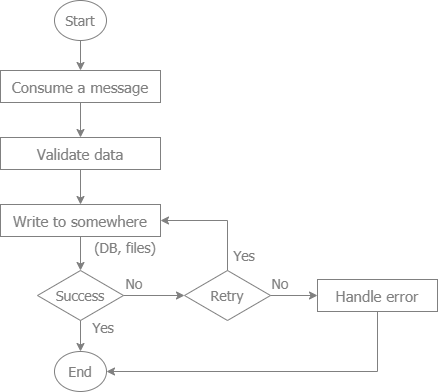 | ||
- The libraries to implement this flow are: | ||
- [mq](https://github.com/core-go/mq) for GOLANG. Example is at [go-nats-sample](https://github.com/project-samples/go-nats-sample) | ||
- [mq-one](https://www.npmjs.com/package/mq-one) for nodejs. Example is at [nats-sample](https://github.com/typescript-tutorial/nats-sample) | ||
|
||
### Use Cases of NATS | ||
#### Microservices Communication: | ||
- <b>Scenario</b>: Facilitating communication between microservices in a distributed system. | ||
- <b>Benefit</b>: Provides low-latency, reliable messaging, ensuring efficient inter-service communication. | ||
 | ||
#### Financial Services: | ||
- <b>Scenario</b>: Enabling real-time transactions and data updates. | ||
- <b>Benefit</b>: Provides reliable and fast message delivery critical for financial applications. | ||
#### Real-Time Data Streaming: | ||
- <b>Scenario</b>: Streaming data in real-time from various sources to data processing systems. | ||
- <b>Benefit</b>: Low latency ensures real-time data processing and analytics. | ||
 | ||
#### Event-Driven Architectures: | ||
- <b>Scenario</b>: Building applications based on event-driven paradigms. | ||
- <b>Benefit</b>: Decouples services, allowing for scalable and maintainable architectures. | ||
#### IoT Messaging: | ||
- <b>Scenario</b>: Handling communication between numerous IoT devices. | ||
- <b>Benefit</b>: Supports lightweight, scalable messaging suitable for IoT environments. | ||
#### Edge Computing: | ||
- <b>Scenario</b>: Managing communication between edge devices and cloud services. | ||
- <b>Benefit</b>: Efficiently handles data transfer and command execution with minimal latency. | ||
|
||
### Comparison of NATS, Kafka, and RabbitMQ | ||
#### NATS: | ||
- <b>Type</b>: Lightweight, high-performance messaging system. | ||
- <b>Use Cases</b>: Microservices communication, IoT messaging, real-time data streaming. | ||
- <b>Delivery Guarantees</b>: At-most-once (standard), at-least-once with JetStream. | ||
- <b>Persistence</b>: Optional (JetStream for persistence). | ||
- <b>Latency</b>: Very low, optimized for speed. | ||
- <b>Scalability</b>: Highly scalable with clustering. | ||
#### Apache Kafka: | ||
- <b>Type</b>: Distributed event streaming platform. | ||
- <b>Use Cases</b>: High-throughput messaging, event sourcing, log aggregation. | ||
- <b>Delivery Guarantees</b>: Configurable (at-least-once, exactly-once). | ||
- <b>Persistence</b>: Durable storage with configurable retention. | ||
- <b>Latency</b>: Higher due to disk persistence. | ||
- <b>Scalability</b>: Highly scalable with partitioned topics. | ||
#### RabbitMQ: | ||
- <b>Type</b>: Message broker. | ||
- <b>Use Cases</b>: Decoupling applications, job queuing, asynchronous communication. | ||
- <b>Delivery Guarantees</b>: At-least-once, exactly-once (with transactions). | ||
- <b>Persistence</b>: Persistent storage of messages. | ||
- <b>Latency</b>: Moderate, designed for reliability. | ||
- <b>Scalability</b>: Scalable with clustering and federation. | ||
|
||
### Key Differences: | ||
- <b>Latency and Performance</b>: NATS offers the lowest latency, Kafka provides high throughput with persistence, RabbitMQ balances reliability and performance. | ||
- <b>Persistence</b>: Kafka and RabbitMQ offer strong persistence guarantees, while NATS focuses on speed with optional persistence. | ||
- <b>Scalability</b>: All three are scalable, but Kafka excels in handling high-throughput event streams, NATS in low-latency scenarios, and RabbitMQ in reliable message delivery. | ||
|
||
### Use Case Suitability: | ||
- <b>NATS</b>: Best for real-time, low-latency communication in microservices and IoT. | ||
- <b>Kafka</b>: Ideal for high-throughput event streaming and log aggregation. | ||
- <b>RabbitMQ</b>: Suitable for reliable message queuing and asynchronous task processing. | ||
|
||
|
||
## Installation | ||
|
||
Please make sure to initialize a Go module before installing common-go/nats: | ||
Please make sure to initialize a Go module before installing core-go/nats: | ||
|
||
```shell | ||
go get -u github.com/common-go/nats | ||
go get -u github.com/core-go/nats | ||
``` | ||
|
||
Import: | ||
|
||
```go | ||
import "github.com/common-go/nats" | ||
import "github.com/core-go/nats" | ||
``` |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -1,6 +1,6 @@ | ||
package nats | ||
|
||
type PublisherConfig struct { | ||
Subject string `mapstructure:"subject" json:"subject,omitempty" gorm:"column:subject" bson:"subject,omitempty" dynamodbav:"subject,omitempty" firestore:"subject,omitempty"` | ||
Connection ConnConfig `mapstructure:"connection" json:"connection,omitempty" gorm:"column:connection" bson:"connection,omitempty" dynamodbav:"connection,omitempty" firestore:"connection,omitempty"` | ||
Subject string `yaml:"subject" mapstructure:"subject" json:"subject,omitempty" gorm:"column:subject" bson:"subject,omitempty" dynamodbav:"subject,omitempty" firestore:"subject,omitempty"` | ||
Connection ConnConfig `yaml:"connection" mapstructure:"connection" json:"connection,omitempty" gorm:"column:connection" bson:"connection,omitempty" dynamodbav:"connection,omitempty" firestore:"connection,omitempty"` | ||
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,53 @@ | ||
package nats | ||
|
||
import ( | ||
"context" | ||
"github.com/nats-io/nats.go" | ||
) | ||
|
||
type SubjectPublisher struct { | ||
Conn *nats.Conn | ||
} | ||
|
||
func NewSubjectPublisher(conn *nats.Conn) *SubjectPublisher { | ||
return &SubjectPublisher{conn} | ||
} | ||
func NewSubjectPublisherByConfig(p PublisherConfig) (*SubjectPublisher, error) { | ||
if p.Connection.Retry.Retry1 <= 0 { | ||
conn, err := nats.Connect(p.Connection.Url, p.Connection.Option) | ||
if err != nil { | ||
return nil, err | ||
} | ||
return NewSubjectPublisher(conn), nil | ||
} else { | ||
durations := DurationsFromValue(p.Connection.Retry, "Retry", 9) | ||
conn, err := NewConn(durations, p.Connection.Url, p.Connection.Option) | ||
if err != nil { | ||
return nil, err | ||
} | ||
return NewSubjectPublisher(conn), nil | ||
} | ||
} | ||
func (p *SubjectPublisher) Publish(ctx context.Context, subject string, data []byte, attributes map[string]string) error { | ||
defer p.Conn.Flush() | ||
if attributes == nil { | ||
return p.Conn.Publish(subject, data) | ||
} else { | ||
header := MapToHeader(attributes) | ||
var msg = &nats.Msg{ | ||
Subject: subject, | ||
Data: data, | ||
Reply: "", | ||
Header: nats.Header(*header), | ||
} | ||
return p.Conn.PublishMsg(msg) | ||
} | ||
} | ||
func (p *SubjectPublisher) PublishData(ctx context.Context, subject string, data []byte) error { | ||
defer p.Conn.Flush() | ||
return p.Conn.Publish(subject, data) | ||
} | ||
func (p *SubjectPublisher) PublishMsg(subject string, data []byte) error { | ||
defer p.Conn.Flush() | ||
return p.Conn.Publish(subject, data) | ||
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -1,7 +1,6 @@ | ||
package nats | ||
|
||
type SubscriberConfig struct { | ||
Subject string `mapstructure:"subject" json:"subject,omitempty" gorm:"column:subject" bson:"subject,omitempty" dynamodbav:"subject,omitempty" firestore:"subject,omitempty"` | ||
Header bool `mapstructure:"header" json:"header,omitempty" gorm:"column:header" bson:"header,omitempty" dynamodbav:"header,omitempty" firestore:"header,omitempty"` | ||
Connection ConnConfig `mapstructure:"connection" json:"connection,omitempty" gorm:"column:connection" bson:"connection,omitempty" dynamodbav:"connection,omitempty" firestore:"connection,omitempty"` | ||
Subject string `yaml:"subject" mapstructure:"subject" json:"subject,omitempty" gorm:"column:subject" bson:"subject,omitempty" dynamodbav:"subject,omitempty" firestore:"subject,omitempty"` | ||
Connection ConnConfig `yaml:"connection" mapstructure:"connection" json:"connection,omitempty" gorm:"column:connection" bson:"connection,omitempty" dynamodbav:"connection,omitempty" firestore:"connection,omitempty"` | ||
} |